ISM
3232 - Intermediate Business Programming.
Chapter 0-1 Student Learning Objectives.
You should be able to:
- Review and describe hardware and software components
and concepts.
- List benefits of using the Java
programming language.
- Briefly describe basic object-oriented (OO) programming
concepts.
- List 6 principles of OO design.
- Distinguish between Java applications
and Java applets.
- Install and configure a Java development environment.
- List and describe the steps needed
to develop a program.
- List 3 principles of good programming style.
- Create, save, compile, and execute a simple Java application
and a Java applet.
- Distinguish between syntax and semantic
(logic) errors.
- Use the system.out.println() object.
Chapter 0.
Review. You should already be familiar with,
and are responsible for, the following concepts in Chapter 0.
- Hardware, CPU, peripherals.
- Software: application, system, programming languages,
compiling.
- Internet and WWW.
What is a computer program?
- Set of instructions that directs the computer's behavior.
- Programming: creating the instructions.
Why Java?
Supports Internet/WWW applications due to:
- simple, robust, secure
- portability = platform independence
- support for distributed applications
- object-oriented = reusability
Object-Oriented (OO) Programming
What is an Object?
- a program module that encapsulates some characteristics
and behavior (data and methods).
Principles of OO Programming.
Divide and Conquer
|
Breaking down a large complex problem
into smaller, more manageable pieces.
|
Encapsulation
|
Each object knows and contains
the data and methods needed to perform its own task.
|
Interface
|
Clearly and explicitly defines
how an object interacts with other objects (public).
|
Information Hiding
|
An object keeps its internal details
hidden and protected from other objects, and only shares what is needed
(private).
|
Generality
|
Designing objects so they can be
re-used and extended.
|
Extensibility
|
Adapting generalized objects to
similar, more specialized tasks.
|
Suggested Review Exercises: #1, 9 (p. 17-18)
Chapter 1.
What is the difference between an application
and an applet,
in Java?
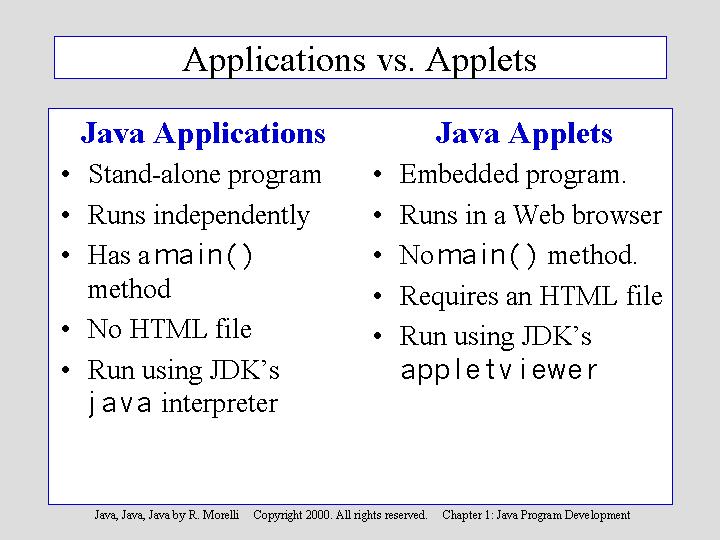
Some Java program components:
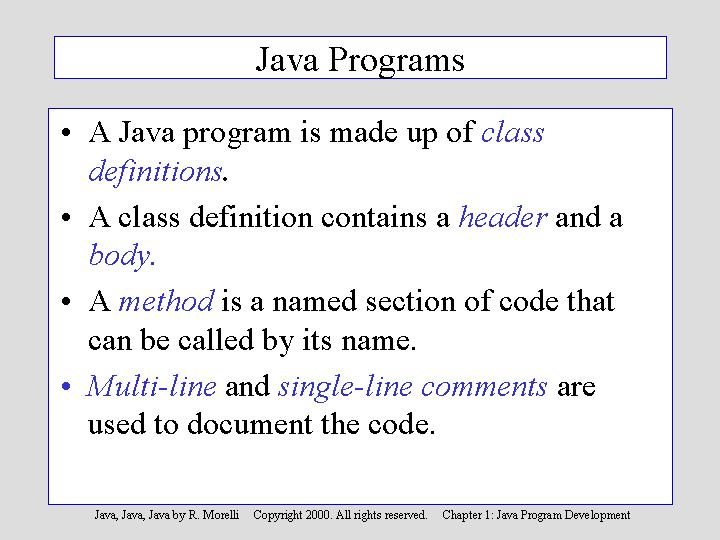
An example of a simple Java application - note the comments,
the class header, and the "main" method. Also, note the right and
left brackets which enclose program blocks.
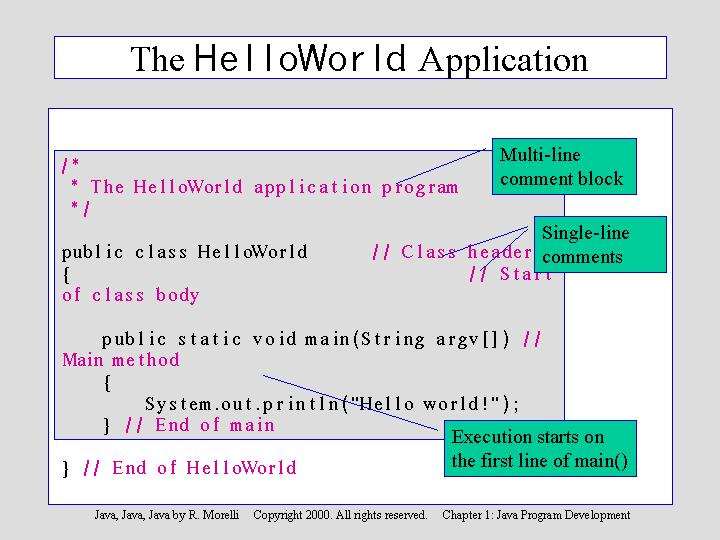
Java applets are more complicated to write than applications.
This is because they are intended to run within a Web browser, and make use
of a Graphical User Interface (GUI). The following example of an applet must
import 2 Java class libraries, java.awt
and java.applet.
This shows Java's ability to frequently re-use existing program modules, and
thus to take advantage of previously developed and tested programs.
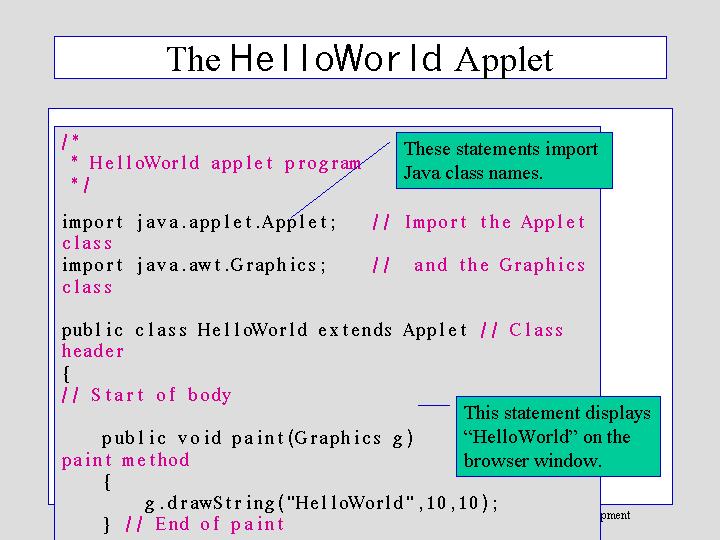
How do I develop a Java application or applet?
There are 3 steps: editing,
compiling, and executing.
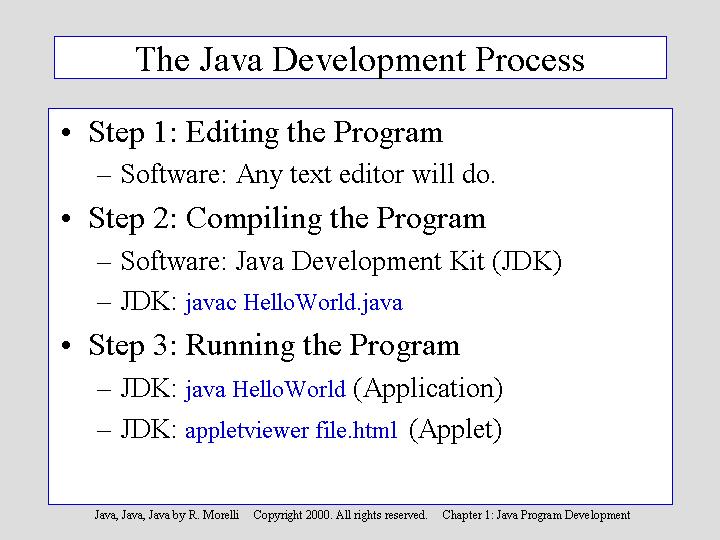
Editing a Java Program
- Software: A text editor (Notepad, Textpad).
- Program source code must be saved in a text
file named <ClassName>.java
where ClassName is the name
of the public class contained in the file.
- Remember: Java class names and file names are
case sensitive.
Compiling a Java Program
- Compilation translates the source program into
Java bytecode.
- Bytecode is platform-independent
- JDK Cmd: javac
HelloWorld.java
- Successful compilation of HelloWorld.java
will create the bytecode class file: HelloWorld.class
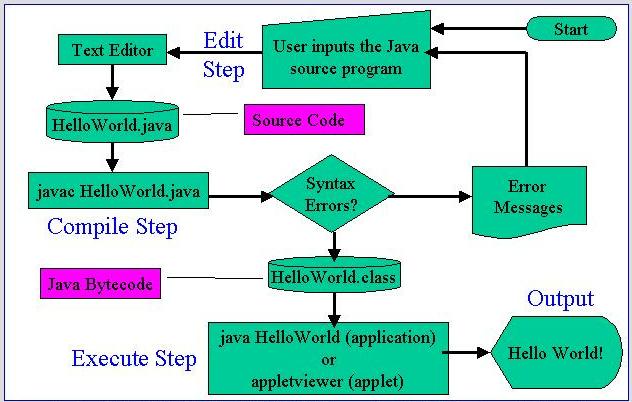
Running (Executing) a Java Application
- The class file (bytecode) is loaded into memory
and interpreted by the Java Virtual Machine (JVM)
- JDK Command:
java HelloWorld
Running (Executing) a Java Applet
- Running an applet requires an additional HTML
file containing an <applet> tag:
Designing Good Programs
- Always: Design before Coding!
- Remember: The sooner you begin to type code,
the longer the program will take to finish.
- Design includes designing:
- classes, data, methods, algorithms
- Design is followed by:
- coding, testing, and revision
Exercise: make a copy of p.
29 in your text, and use it as a reference every time you sit down to develop
a new program.
The Software Development
Process
- Problem Specification
(understand the problem!)
- Problem Decomposition
(break it down into pieces!)
- Design Specification
- Data, Methods, and Algorithms
- Coding into Java
- Testing, Debugging, and Revising
To Specify the Problem, ask yourself
(and answer) these 3 questions:
- What exactly is the problem to be solved?
- What information will the program be given as input?
- What results will the program be expected to produce
(output)?
To Decompose
the Problem
- Divide the problem into parts to make the solution
more manageable.
- Divide-and-Conquer
repeatedly until subproblems are simple to solve.
- In Object-Oriented Design, each object will
solve a subproblem.
To Design,
ask and answer these questions for each object:
- What subtask(s) will the object perform?
- What information will it need to perform
its task (data)?
- Which actions will it use to process the
information (methods)?
- What interface will it present to other
objects (public)?
- What information will it hide from other
objects (private)?
Example: Design Specification for the Rectangle class
-
Note how each of the above
questions is answered.
- An instance variable is a memory location
used for storing the information needed.
- A public method is a block of code
used to perform a subtask or manipulation needed.
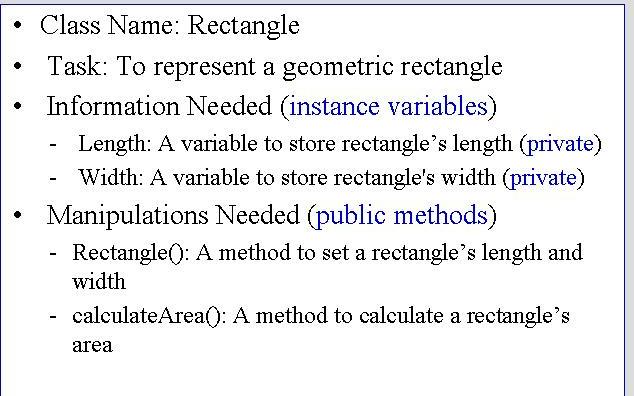
More Design: Data, Methods, and
Algorithms
- What type of data will be used to represent the
information needed by the rectangle?
- How will each method carry out its appointed task?
Method Design
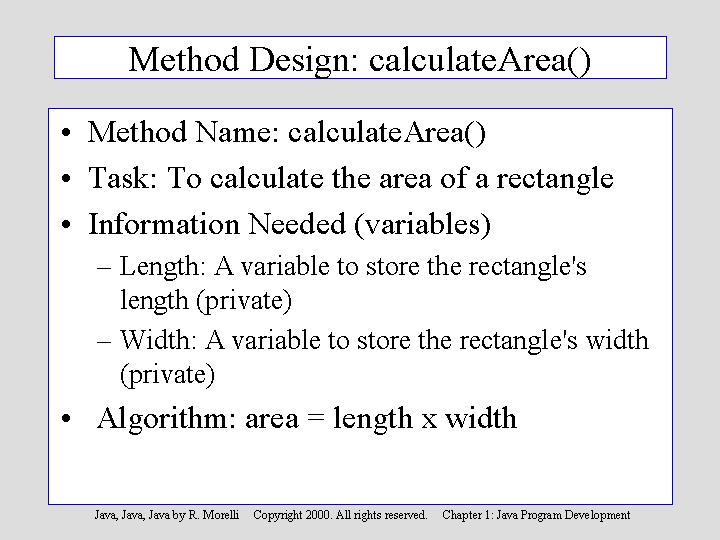
Coding into Java: Always Use
Stepwise Refinement
- Stepwise Refinement is the right
(and easiest!) way to code.
- Code small stages at a time
- Compile and test in between.
- Errors are caught earlier
Exercise: Take the Stepwise Refinement Viewlet Tutorial.
- Follow Syntax rules
- Syntax is the set of rules that determine
whether a particular statement is correctly formulated - caught by
compiler
- Prevent Semantic (logic) errors
- Semantics: the meaning (effect on
the program) of each Java statement - not caught by compiler
Testing, Debugging, and Revising
- Coding, testing, and revising a program is an iterative
process.
- The java compiler catches syntax errors,
producing error messages.
- The programmer must test thoroughly for semantic
errors (logic errors).
- Semantic errors are errors which manifest themselves
through illogical output or behavior.
- Errors are corrected in the debugging phase
Writing Readable Programs
Good Programming Style
- Readability.
- Code should be well-documented and easy to understand.
- Clarity.
- Conventions should be followed and convoluted
code avoided.
- Flexibility.
- Code should be designed for easy maintenance
and change.
Simple non-graphical Input and Output
in Java
-
A source or destination for data is considered a stream
of characters (bytes).
-
These streams are handled through pre-defined classes
in the
java.io
class library.
- java.io.printstream
is a class in the java.io library
that includes methods such as print()
and println()
- System.out is
an object in Java's system
library that can invoke print()
and println()
Example in HelloWorld (see above):
System.out.println("Hello
World!");
Also see example on p. 40, OldMacDonald.java
class.
Qualified Names.
The dot notation tells the Java compiler where to find the "referent"
(the leftmost item in the name). The qualifiers may refer to packages, libraries,
classes, etc. depending upon the context in which they are used.
System.out.println() refers
to the Java System class
library, the out object,
and the println() method.
Suggested Exercises for Chapter 1: All self-study
exercises, tutorial viewlets, practice quizzes from the text Web site.