Implementing Shopping Carts
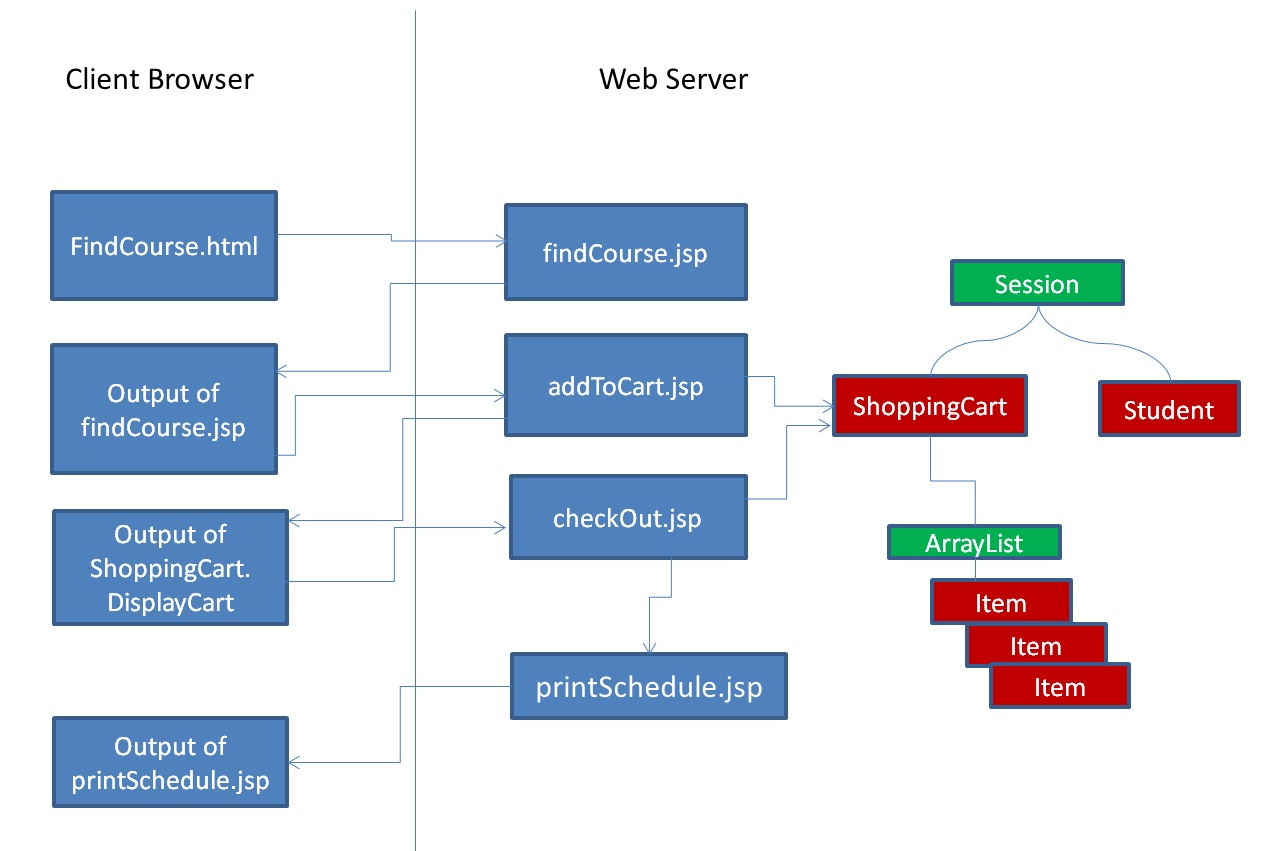
Part 1 - Create the java classes to perform the function of the
cart and an item in the cart
- Under Source Packages, create
package for your classes, in my example it is dbutility
- Create a Shopping cart class
- This class should have an ArrayList object as a class variable
to server as the container for the cart
- Constructor that creates an empty cart (ArrayList)
- Method to add an item from the cart
- add method should handle duplicate objects
- Method to remove an item from the cart
- Method to display the carts contents
- this method could provide a column with a link to a remove jsp
ShoppingCart.java
package registrarbeans;
import java.io.IOException;
import java.util.ArrayList;
import javax.servlet.jsp.JspWriter;
import java.sql.*;
/**
*
* @author Mark Pendergast
*/
public class ShoppingCart {
ArrayList<Item> itemlist = new
ArrayList<Item>(); // list of Items in the cart
java.text.DecimalFormat currency = new
java.text.DecimalFormat("$ #,###,##0.00");
public ShoppingCart()
{
}
public void empty()
{
itemlist.clear();
}
//
// add an item to the cart
// if its there already, just update the upc
//
public void add(Item anitem)
{
for(int i = 0; i < itemlist.size(); i++)
{
Item item = itemlist.get(i);
if(anitem.id == item.id) // already in
the cart?
{
item.quantity += anitem.quantity;
// yes, just update the quantity
return;
}
}
itemlist.add(anitem); // no, add it as a new
item
}
//
// remove an item with a given id from the shopping
cart
//
public void remove(int id)
{
for(int i = 0; i < itemlist.size(); i++)
{
Item item = itemlist.get(i);
if(id == item.id) // item in the cart?
{
itemlist.remove(i); // remove it
break;
}
}
}
//
// Display the current contents of the cart as an html
table
//
public void display(JspWriter out) throws IOException
{
//
// start the table and output the header
row
//
out.println("<h3>Cart
contents</h3>");
out.println("<table border=1>");
out.println("<tr><th>ID</th><th>Name</th><th>Price</th><th>Quantity</th><th>Total</th></tr>");
double total = 0;
//
// output one item at a time from the
cart, one item to a row table
//
for(int i = 0; i < itemlist.size();
i++)
{
Item item = (Item)itemlist.get(i);
out.println("<tr><td>"+item.id+"</td>"+
"<td>"+item.name+"</td>"+
"<td align=right>"+ currency.format(item.price)+"</td>"+
"<td align=right>"+ item.quantity+"</td>"+
"<td align=right>"+
currency.format(item.price*item.quantity)+"</td>"+
"<td align=center><A
href='removeItemFromCart.jsp?id="+item.id+"'>remove</A></TD></tr>");
total += item.price*item.quantity;
}
//
// add summary information (total, tax,
grand total)
//
out.println("<tr><td
colspan = 4>Total purchase</td>");
out.println("<td
align=right>"+currency.format(total)+"</td></tr>");
out.println("<tr><td
colspan = 4>Sales tax @6%</td>"+
"<td align=right>"+
currency.format(total*.06)+"</td></tr>");
out.println("<tr><td
colspan = 4>Amount due</td>"+
"<td align=right>"+
currency.format(total*1.06)+"</td></tr>");
out.println("</table>");
out.println("<a
href='checkOut.jsp'><input type='Button'
value='Register'/></A><br/>");
}
public int checkOut(String url, String studentid)
{
int result = 0; // tally the
classes added
try{
// open a connection
Connection con =
null;
Class.forName("com.microsoft.sqlserver.jdbc.SQLServerDriver"); //
load the driver
con =
DriverManager.getConnection(url);
//
// add entry to enroll table for each item
//
PreparedStatement prep =
con.prepareStatement("Insert into enroll (StudentID, CRN) values
(?,?)");
for(int i = 0; i <
itemlist.size(); i++)
{
Item item
= itemlist.get(i);
prep.setString(1, studentid);
prep.setInt(2, item.id);
result +=
prep.executeUpdate();
}
itemlist.clear(); //empty
the cart
prep.close();
con.close();
}
catch(Exception ex)
{
// unable to
close the prepared statement
}
return result;
}
}
- Create a class that holds information on an item that goes into
the cart
- Class should have all the data fields to represent the item
when it is displayed for the user
- Class should have a constructor to create an object (a default
constructor as well)
- Class should have a toString as well.
- Other methods as needed by JSPs
Item.java
package registrarbeans;
/**
*
* @author Mark Pendergast
*/
public class Item {
int id = 0;
String name = "";
int quantity = 0;
double price = 0;
public Item()
{
}
//
// mutator methods
//
public void setId(int n)
{
id = n;
}
public void setName(String s)
{
name = s;
}
public void setQuantity(int n)
{
quantity = n;
}
public void setPrice(double n)
{
price = n;
}
//
// Accessor methods
//
public int getId()
{
return id;
}
public String getName()
{
return name;
}
public int getQuantity()
{
return quantity;
}
public double getPrice()
{
return price;
}
@Override
public String toString()
{
return id+" "+name+" "+quantity +" @ "+price;
}
}
Part 2 - Create a Search form and corresponding JSP that finds the
objects and provides "add to cart" links
- Form should accept search parameters
- Form should have javascript to validate parameters
FindCourse.html
-->
<!DOCTYPE html>
<html>
<head>
<title></title>
<meta
http-equiv="Content-Type" content="text/html; charset=UTF-8">
</head>
<body>
<div>
<form name="findCourse"
method="post" action="findCourseWithAddToCart.jsp" >
<Label for="course">Course</Label><input type ="text"
id="course" name="course" size="12" /> <br/>
<Label for="title">Title</Label><input type ="text"
id="title" name="title" size="30" /> <br/>
<Label for="instructor">Instructor</Label><input type
="text" id="instructor" name="instructor" size="30" /> <br/>
<Label for="seatsleft">Seats left greater
than</Label><input type ="text" id="seatsleft"
name="seatsleft" size="6" /> <br/>
<input type="submit" value="find courses" /> <input
type="reset" value="clear"
/>
</form>
</div>
</body>
</html>
- JSP should perform a search of the database with parameters from
the search form
- Output should be formatted as an HTML table with an extra column
that links to the add to cart jsp
- Be sure to fix data in the URL link
- Optionally you can create an inline frame to show the shopping
cart, just add a target to the link and an iframe tag, both refering to
the same name
findCourseWithAddToCart.jsp
%--
Document : findCourse
Created on : Oct 18, 2012, 2:37:49 PM
Author : mpenderg
--%>
<%@page contentType="text/html" pageEncoding="UTF-8"%>
<%@page import="java.sql.*"%>
<!DOCTYPE html>
<html>
<head>
<meta
http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Courses</title>
</head>
<body>
<h1>Courses that
match</h1>
<%
String course =
"";
String title =
"";
String
instructor = "";
String
seatsleftStr = "0";
if(request.getParameter("course") != null)
course = request.getParameter("course");
if(request.getParameter("title") != null)
title = request.getParameter("title");
if(request.getParameter("instructor") != null)
instructor = request.getParameter("instructor");
if(request.getParameter("seatsleft") != null)
seatsleftStr= request.getParameter("seatsleft");
int seatsleft =
0;
try{
seatsleft
= Integer.parseInt(seatsleftStr);
}
catch(Exception ex1)
{
out.println("Invalid
value for seats left, using 0<br/>");
}
//
// database work
//
try{
// open a connection
Connection con =
null;
Class.forName("com.microsoft.sqlserver.jdbc.SQLServerDriver"); //
load the driver
con =
DriverManager.getConnection("jdbc:sqlserver://rubble.student.ad.fgcu.edu:1433;databaseName=sp12ism3232x;user=s12ISM3232;password=s12ISM3232;");
// create the sql command
PreparedStatement prep = con.prepareStatement("Select * from
courseschedule where course like ? and title like ? and instructor like
? and seatsleft >= ?");
prep.setString(1,course+"%");
prep.setString(2,"%"+title+"%");
prep.setString(3,instructor+"%");
prep.setInt(4,seatsleft);
ResultSet rs =
prep.executeQuery();
%>
<table border ="1">
<tr>
<td>CRN
</td>
<td>Course</td>
<td>Title
</td>
<td>Credits
</td>
<td>Instructor
</td>
<td>Max
seats</td>
<td>Seats
left</td>
<td>College
</td
</tr>
<%
// process
results one row at a timne
while(rs.next())
{
out.println("<tr>");
out.println("<td>"+rs.getString(1)+"</td>");
out.println("<td>"+rs.getString(2)+"</td>");
out.println("<td>"+rs.getString(3)+"</td>");
out.println("<td>"+rs.getString(4)+"</td>");
out.println("<td>"+rs.getString(5)+"</td>");
out.println("<td>"+rs.getString(6)+"</td>");
out.println("<td>"+rs.getString(7)+"</td>");
out.println("<td>"+rs.getString(8)+"</td>");
out.println("<td><a
href='deleteCourse.jsp?CRN="+rs.getString(1)+"'><input
type='button' value='delete'/></A></td>");
String name
=rs.getString(2)+" "+rs.getString(3);
name = java.net.URLEncoder.encode(name, "UTF-8"); // fix name
into a url ok
out.println("<td><a
href='addItemToCart.jsp?id="+rs.getString(1)+"&name="+name+"&quantity=1&price=9.95"+
"'><input type='button' value='add'/></A></td>");
out.println("</tr>");
}
%>
</table>
<%
prep.close();
con.close();
}
catch(Exception ex)
{
out.println("Sorry, the system is unavailable<br/>");
out.println(ex.toString()+"<br/>");
}
%>
</body>
</html>
Part 3 - Create an add to cart JSP that responds to the link
generated in part 2
- This jsp should access the shopping cart through the session
varaible, if a cart does not exist, then create one and add it to the
session.
- Then the jsp should create a object to hold the items data (in
this case an Item object)
- Finally, add the object to the cart and tell the cart to display
itself.
addItemToCart.jsp
<%--
Document : addItemToCart
Created on : Oct 24, 2012, 1:46:32 PM
Author : Mark Pendergast
--%>
<%@page contentType="text/html" pageEncoding="UTF-8"%>
<%@page
import="registrarbeans.*"%>
<%@page errorPage = "errorPage.jsp"
%>
<jsp:useBean id="item"
class="registrarbeans.Item" scope = "request" />
<jsp:useBean id="cart"
class="registrarbeans.ShoppingCart" scope ="session" />
<jsp:setProperty name="item"
property="*" />
<!DOCTYPE html>
<html>
<head>
<meta
http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Shopping
Cart</title>
</head>
<body>
<%
//
// set the session's inactive interval
//
session.setMaxInactiveInterval(1800);
// 30 minutes
//
// now add the item to the cart
//
synchronized(session) // lock the session
{
cart.add(item); // cart uses ArrayList which is not thread safe so we
locked
cart.display(out); // tell the cart to send its contents to the browser
}
%>
</body>
</html>
Part 4 - Create JSP to handle removing
an item from the cart
- If the display method of your shopping cart provides a
remove link, then you'll have to create a jsp to respond to this.
- This jsp should first access the cart, if its not available, then
create an empty one
- If it is available, then call the carts remove method with data
provided on the form parameter
- Tell cart to redisplay itself
removeItemFromCart.jsp
<%@page contentType="text/html" pageEncoding="UTF-8"%>
<jsp:useBean id="cart"
class="registrarbeans.ShoppingCart" scope ="session" />
<%@page errorPage = "errorPage.jsp"
%>
<!DOCTYPE html>
<html>
<head>
<meta
http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Remove</title>
</head>
<body>
<%
session.setMaxInactiveInterval(1800); // make session expire after 30
minutes
//
// Remove the
item
String idstr =
request.getParameter("id");
try
{
int id =
Integer.parseInt(idstr);
synchronized(session) // lock the
session
{
cart.remove(id);
}
}
catch(Exception
ex)
{
out.println("Error: "+ ex.toString()+ "<br/>");
}
cart.display(out);
%>
</body>
</html>
Part 5 - Check out
- Finally, you'll want to create some mechanism to allow the user
to checkout the shopping cart.
- You'll need to create a jsp that handles the checkout link output
when your shoppng cart is displayed, in my case this is
RegisterCourses.jsp
- That jsp should do the following:
- Verify a user is logged in
- Create a connection to the database
- get the shoppnig cart from the session
- create a db connection
- ask the shopping to perform a checkout funtion, passing the
login info and connection
- the shopping cart will then create the necessary databse
records, then empty itself.
checkOut.jsp
<%@page contentType="text/html" pageEncoding="UTF-8"%>
<jsp:useBean id="cart"
class="registrarbeans.ShoppingCart" scope ="session" />
<jsp:useBean id="student"
class="registrarbeans.Student" scope="session" />
<%@page errorPage = "errorPage.jsp"
%>
<!DOCTYPE html>
<html>
<head>
<meta
http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Checkout</title>
</head>
<body>
<%
session.setMaxInactiveInterval(1800); // make session expire after 30
minutes
if(student.isLoggedIn())
{
synchronized(session)
{
cart.checkOut("jdbc:sqlserver://rubble.student.ad.fgcu.edu:1433;databaseName=sp12ism3232x;user=s12ISM3232;password=s12ISM3232;",
student.getId() );
}
}
else
response.sendRedirect("login.html");
%>
</body>
</html>
Part 6 - Displaying the receipt (course schedule)
- to do this you'll need to create a jsp that finds the records
added during checkout and formats them as a receipt., in this case, a
course schedule
- verify the user is logged in
- use the login information to construct a query for the records
you want.
- execute and display the query.
printSchedule.jsp
<%@page contentType="text/html" pageEncoding="UTF-8"%>
<jsp:useBean id="student" class="registrarbeans.Student"
scope="session" />
<%@page errorPage = "errorPage.jsp" %>
<%@page import="java.sql.*"%>
<!DOCTYPE html>
<html>
<head>
<meta
http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Course
schedule</title>
</head>
<body>
<h2>Courses</h2>
<%
if(!student.isLoggedIn())
response.sendRedirect("login.html");
else
{
try{
//
load the driver and create the connection
Connection con = null;
Class.forName("com.microsoft.sqlserver.jdbc.SQLServerDriver"); //
load the driver
con
=
DriverManager.getConnection("jdbc:sqlserver://rubble.student.ad.fgcu.edu:1433;databaseName=sp12ism3232x;user=s12ISM3232;password=s12ISM3232;");
PreparedStatement prep = con.prepareStatement("select
courseSchedule.crn, courseSchedule.title, courseSchedule.instructor
from courseschedule, enroll where enroll.studentid = ? and
courseschedule.crn =
enroll.crn");
prep.setString(1,student.getId());
ResultSet rs = prep.executeQuery();
out.println("<table>");
out.println("<tr><th>CRN</th><th>Title</th><th>Instructor</th></tr>");
while(rs.next())
{
out.println("<tr>");
out.println("<td>"+rs.getString(1)+"</td>");
out.println("<td>"+rs.getString(2)+"</td>");
out.println("<td>"+rs.getString(3)+"</td>");
out.println("</tr>");
}
out.println("</table>");
prep.close();
con.close();
}
catch(Exception ex)
{
out.println("Sorry, system is unavailable");
out.println(ex.toString());
}
}
%>
</body>
</html>