ISM
3232 - Intermediate Programming.
Chapter 6 - Loops (Repetition)
|
Student Learning Objectives. You
should be able to:
- Design and implement loop structures to solve programming problems
with repetition in Java.
- Specify the differences between loop structures.
- Prevent infinite loops.
- Distinguish between counting and conditional loops.
- List and demonstrate principles of loop design.
- Describe and demonstrate design principles of modularity and localization.
- Explain the role of structured programming in OO program design.
- Use TextArea
|
Index:
|
Review: Flow-of-Control - Repetition
Structures
- Repetition structure: a control structure
that repeats a statement or a sequence of statements.
- Many programming tasks require a repetition structure.
You
are already familiar with the purpose and varieties of loops from your study
of other programming languages such as Visual BASIC or C. This chapter will
be largely review of numerous looping concepts. What will be new is how loops
are implemented in Java.
For
a simple example, what if we wanted to print "Hello" 100 times?
Solution:
Write a method containing 100 println() statements,
or (much
better)
write a method
that contains 1 println(), then place it inside a loop structure:
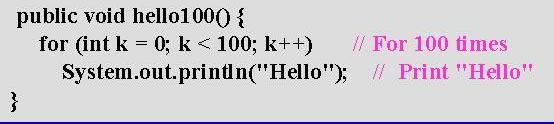
What defines the loop structure?
- It begins with for and ends
with a semi-colon;
- How many times? 100
- This is an example of a counting loop.
Counting Loop
- If number of iterations is known, use a counting
loop.
For example, here is the pseudocode for:
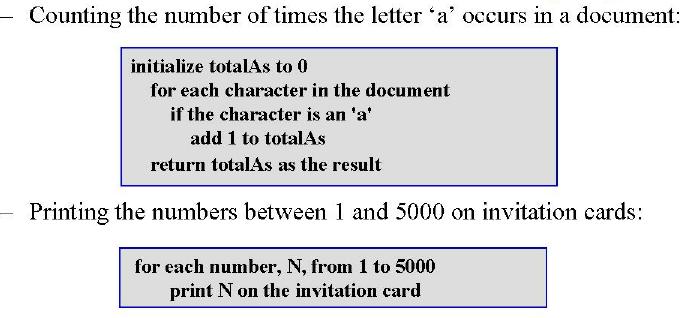
- The for statement is used in counting
loops.
- Each loop must have an initializer, a loop
entry condition, and an updater, that ensures that the loop will
eventually end
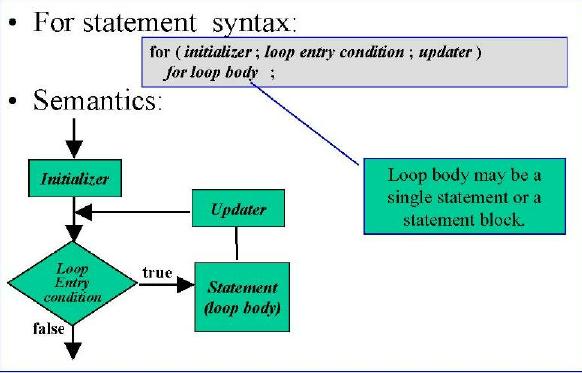
Loop Bounds
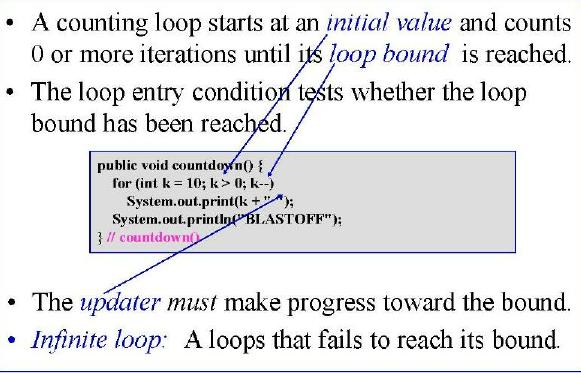
Not
only must an updater exist within the body of the loop, but it must be updated
such that eventually it will end the loop.
Infinite Loop Examples (what
needs to be changed in each?)
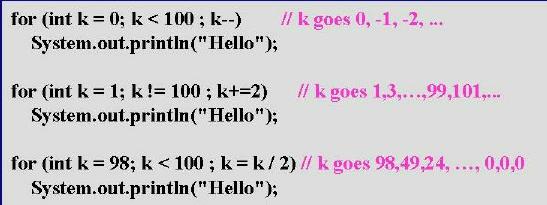
In
each case the updater fails to make progress toward the bound and the loop entry
condition never becomes false.
Loop
Indentation. Indenting properly is extremely important
for readability and debugging purposes, even though the compiler doesn't recognize
it. These examples all appear the same to the compiler, but the first most clearly
illustrates to the reader what is intended.
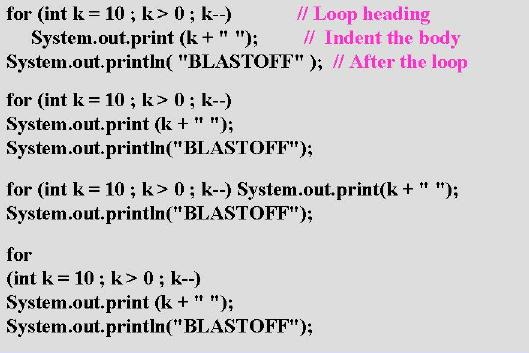
Loop Variable Scope
If k is declared within the for statement,
it cannot be used after the for
statement:

If k is declared before the for statement,
it can be used after the for
statement:
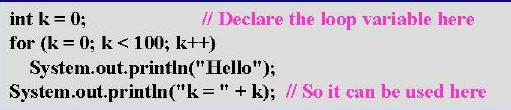
Compound Loop Body
- Compound statement or block: a sequence of statements enclosed within braces,
{...}.
- For loop body: Can be a simple
or compound statement.
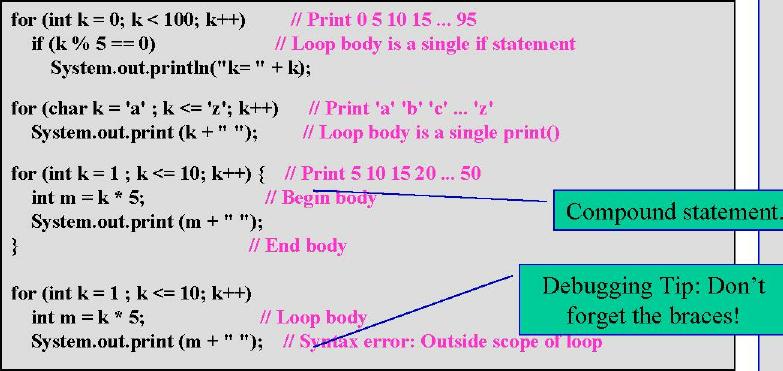
Nested Loops
- Suppose you wanted to print the following table:
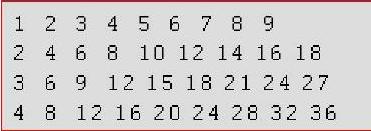
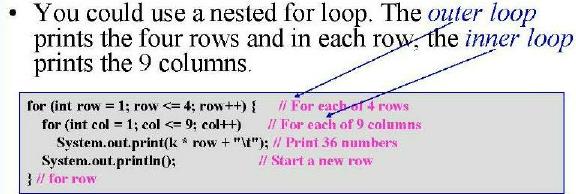
Another Example: Printing a pattern
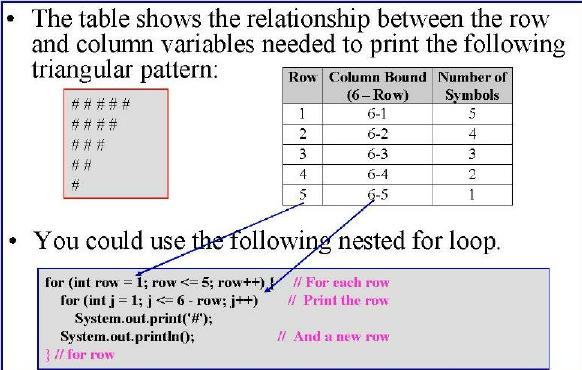
Another Example: Car
Loan Table
- Design a program to print a table for the total
cost of car financing options.
Car Loan Interest Rate
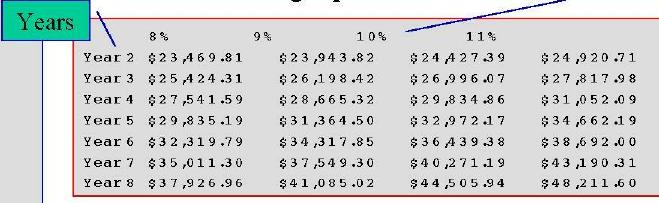
Implementation: CarLoan
Class
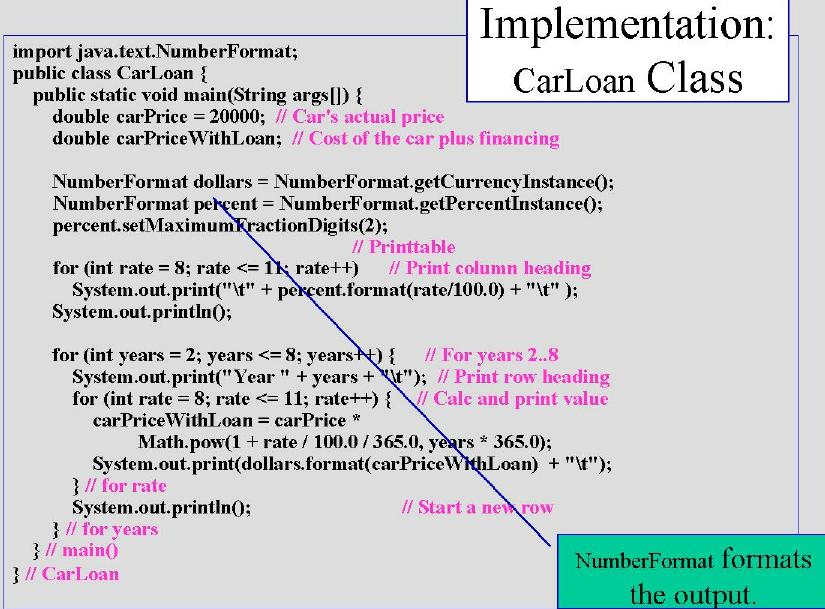
Conditional Loops: While .. , Do
.. While
- When the number of iterations is unknown, we can
use a conditional loop. Examples
in pseudocode:
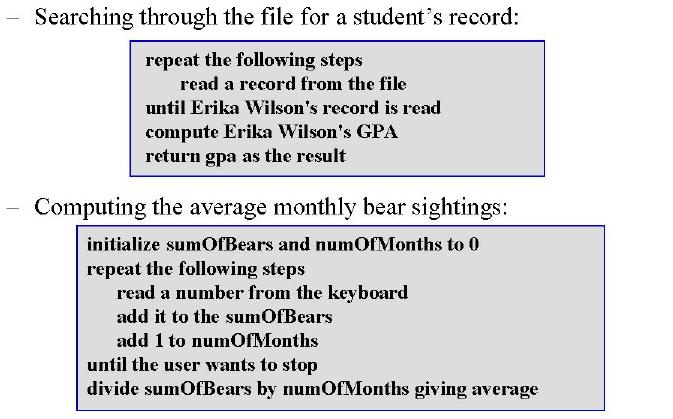
The While Structure
Effective Design: Loop structure.
- A loop structure must include an initializer, a boundary condition, and
an updater. The updater should guarantee that the boundary condition is reached,
so the loop will eventually terminate.

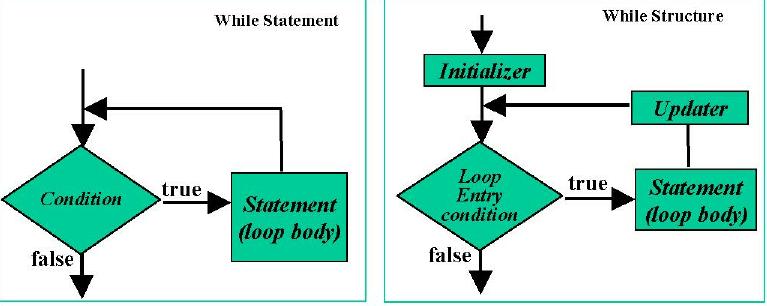
Example while
: Computing Average
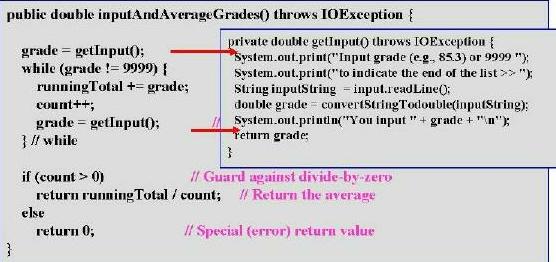
Design Principles: Modularity
and Localization
The
Computing Average example above demonstrates design principles of Modularity
and Localization, by using separate methods for the input and averaging tasks.
We can then call getInput() when needed, rather than repeat the code in multiple
places.
Computing Average: Main Program
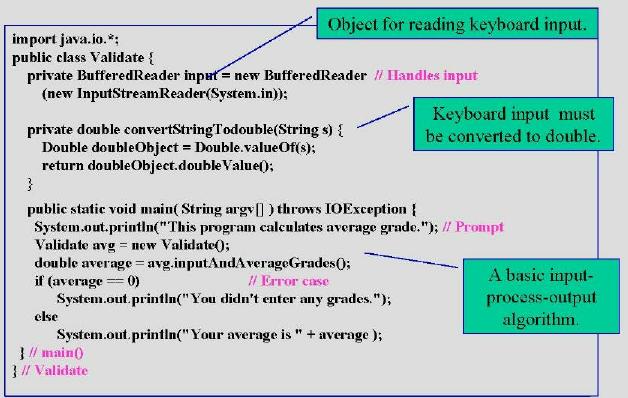
The Do-While
Structure
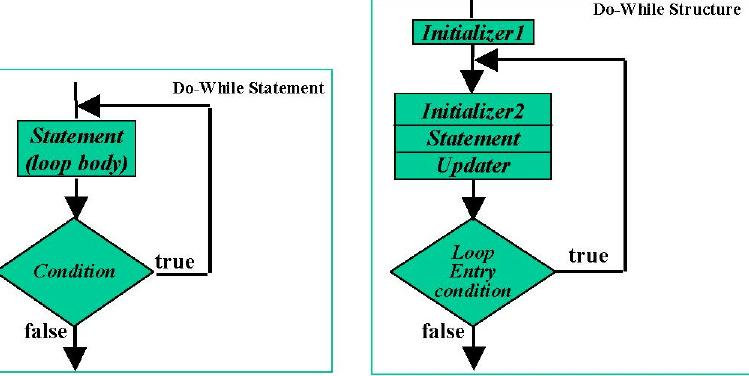
Example:
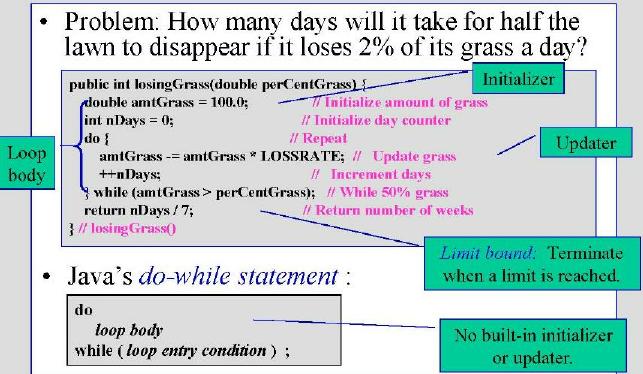
Data Validation:
Example
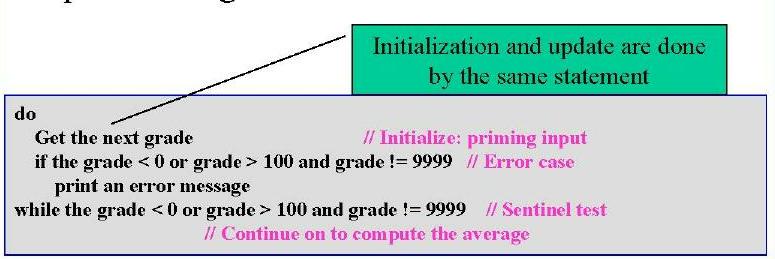
Example: Modifications to CyberPet
- Problem: Modify CyberPetApplet to present an animation of the pet’s eating
behavior.
- Class Design: No changes in CyberPet itself are necessary.
- Algorithm Design: Use a loop to iterate among a set of images that show
CyberPet chewing its food:
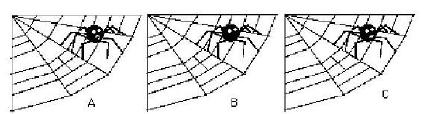
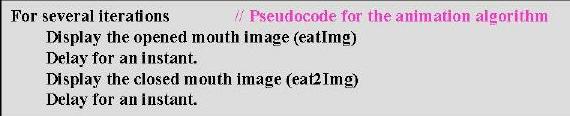
Modified CyberPet Applet
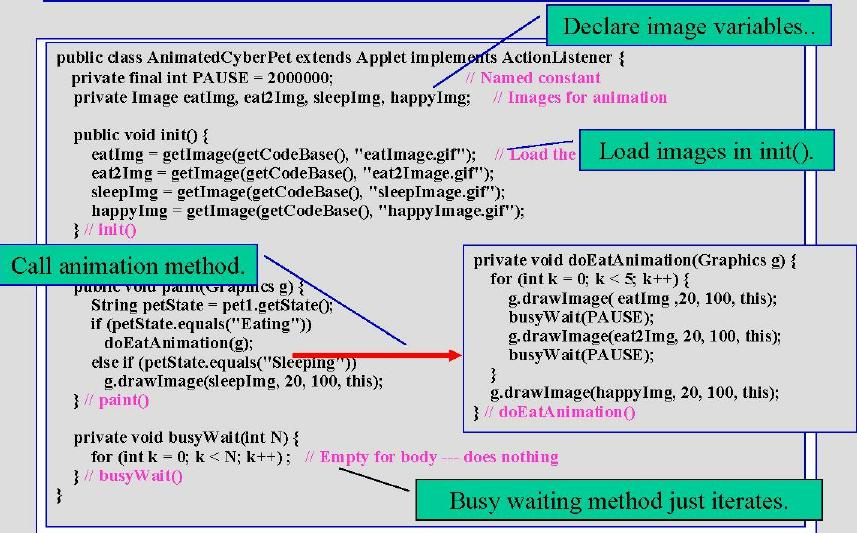
Principles of Loop
Design
- A counting loop can be used if you know
in advance how many iterations are needed. The for statement is used
for counting loops.
- A while structure should be used if the
loop body may be skipped entirely. The while statement is used.
- A do-while structure should be used only
if a loop requires one or more iterations. The do-while-statement should
be used.
- The loop variable, which is used to specify
the loop entry condition, must be initialized to an appropriate value
and updated on each iteration.
- A loop's bound, which may be a count,
a sentinel, or, more generally, a conditional bound, must be
correctly specified in the loop-entry expression, and progress toward it must
be made in the updater.
- An infinite loop may result if either the
initializer, loop-entry expression, or updater expression is not correctly
specified.
Structured
Programming
- Structured programming: uses a small set
of predefined control structures.
- Sequence --- The statements in a program
are executed in sequential order unless their flow is interrupted by one of
the following control structures.
- Selection--- The if, if/else,
and switch statements are branching statements that allow choice by
forking of the control path into two or more alternatives.
- Repetition --- The for, while,
and do-while statements are looping statements that allow the program
to repeat a sequence of statements.
- Method Call --- Invoking a method transfers
control temporarily to a named method. Control returns to the point of invocation
when the method is completed.
Structured Programming Constructs
- No matter how large or small a program is, its flow of control can be built
as a combination of these four structures.
- Note that each structure has one entry and one exit.
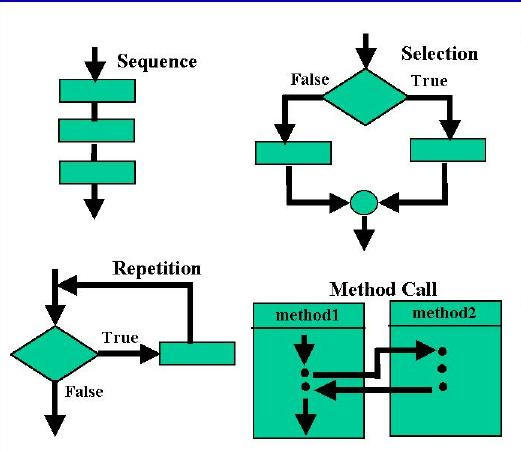
Debugging Problem: Structured vs. Unstructured
Code
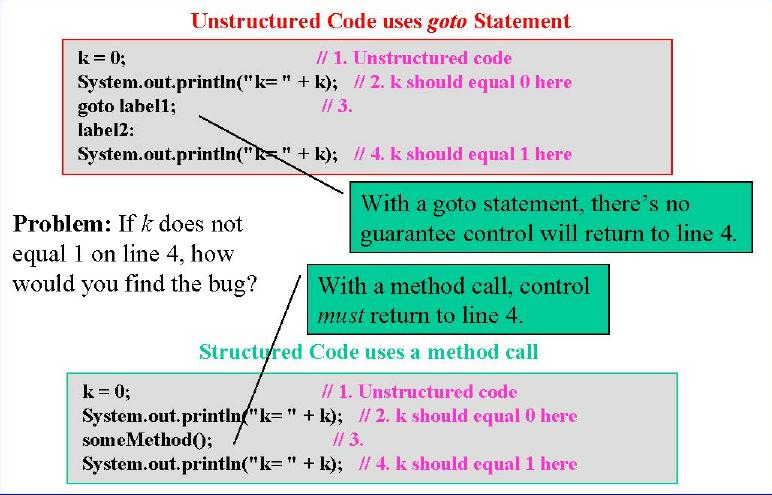
Preconditions and Postconditions
- A precondition is a condition that must
be true before some segment of code is executed.
- A postcondition is a condition that must
be true after some segment of code is executed.
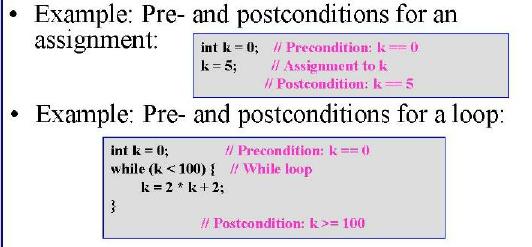
Using Preconditions and Postconditions
- Design stage: Using pre- and postconditions
helps clarify the design and provides a precise measure of correctness.
- Implementation and testing stage: Test data
can be designed to demonstrate that the preconditions and postconditions hold
for any method or code segment.
- Documentation stage: Using pre- and postconditions
to document the program makes the program more readable and easier to modify
and maintain.
- Debugging stage: Using the pre- and postconditions
provides precise criteria that can be used to isolate and locate bugs. A method
is incorrect if its precondition is true and its postcondition is false. A
method is improperly invoked if its precondition is false.
Design/Programming Tips
- Preconditions and postconditions
are an effective way of analyzing the logic of your program's loops and methods.
They should be identified during the design phase and used throughout development,
testing and debugging , and included in the program's documentation.
- Develop your program's documentation at
the same time that you develop its code.
- Acquire and use standard programming techniques
for standard programming problems. For example, using a temporary variable
to swap the values of two variables is an example of a standard technique.
- Use methods wherever appropriate in your
own code to encapsulate important sections of code and thereby reduce complexity.
From the Java Library: TextArea
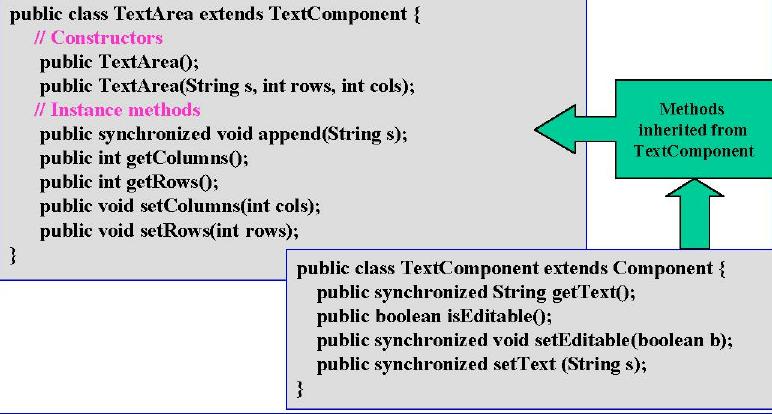
Key terms :
Suggested Exercises : 2, 3, 4, 7, 8, 9