ISM
3232 - Intermediate Business Programming.
Chapter 5 Student Learning Objectives.
You should be able to:
- Explain the difference
between boolean, numeric, and character data.
- Evaluate boolean expressions
and logical operations.
- Evaluate integer, floating
point, and mixed arithmetic expressions.
- Evaluate pre- and post-increment
and decrement operations.
- Use and explain the benefits
of class constants.
- Explain and use static
class variables and methods.
- Perform data conversions in Java.
- Demonstrate your understanding
of information hiding.
- Use Java's math and NumberFormat
classes to solve programming problems and to format output.
- Demonstrate program design that is robust, maintainable,
extensible, and modular.
Review: Data Types
The concept of different data types and how they are stored and
represented in memory is familiar from previous programming classes. We will
focus on how various types of data, and arithmetic and relational expressions,
are implemented in Java. This chapter demonstrates several examples of these
implementations, as well as how they can be used to create good programming
practices.
Programming = Representation + Action
Representation:
- Finding a way to look at a problem.
- Seeing the problem as related to a known problem.
- Being able to divide the problem into smaller solvable
problems.
- Choosing the right kinds of objects and structures.
Action:
- The process of taking well-defined steps to solve a
problem.
- Given a particular way of representing the problem,
what steps must we take to arrive at its solution?
Review: Boolean Data and Operators
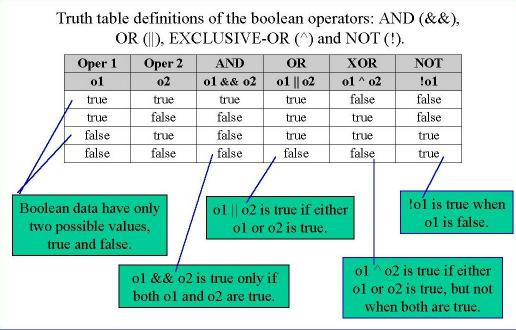
Boolean Operator Precedence
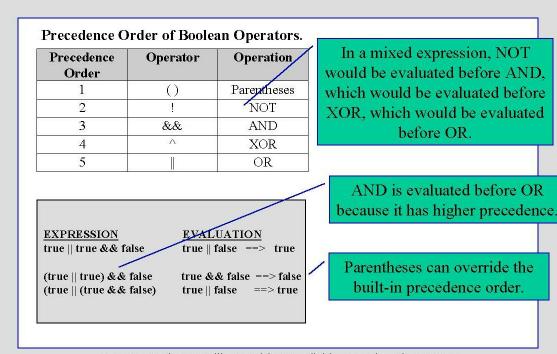
The Boolean-based CyberPet Model is impractical,
because it is not easily extensible:
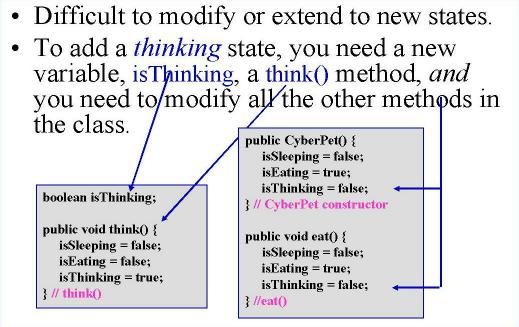
Design Principles
Effective Design: Scalability Principle. A well-designed
model or representation should be easily extensible. It should be easy
to add new functionality to the model.
Effective Design: Modularity Principle. A well-designed
representation will allow us to extend the model without having to modify existing
design methods.
We'll now review numeric data types and then
see how to re-design the CyberPet using a different data type that allows it
to be more extensible and modular.
Numeric Data Types
- Each bit can represent two values.
- An n-bit quantity can represent 2n
values. 28 = 256 possible values.
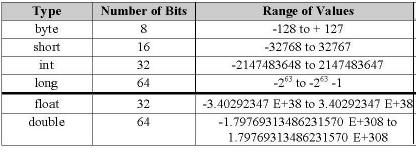
Effective Design: Platform Independence. In Java,
a data type’s size is part of its definition and therefore remains consistent
across all platforms.
Numeric Data: Limitations
Numeric types are representations of number systems,
so there are tradeoffs:
- A finite number of integers can be represented.
- A finite number of values between 1.11 and 1.12, for
example.
- Round-off errors
- Precision
Debugging Tip: A round-off error is the
inability to represent certain numeric values exactly. A
float can have at most 8 significant digits. So 12345.6789 would be rounded
off to 12345.679.
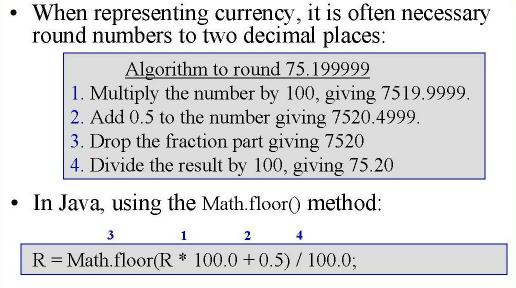
Debugging Tip: Significant Digits. In using numeric
data the data type you choose must have enough precision to represent the values
your program needs.
Review: Standard Arithmetic Operators
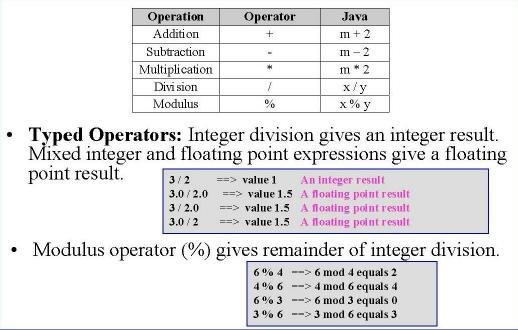
Promotion:
- Converting one data type to another.
- If an expression contains both integer and floating
point types, the integers are promoted to floating point before the operation
takes place.
- In general, when 2 different types are in an expression,
the smaller type is promoted (converted) to the larger, and then the expression
is evaluated.
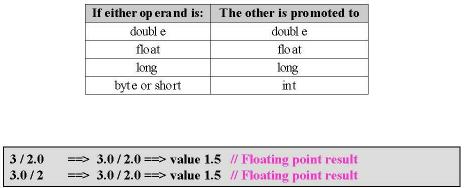
Review: Numeric Operator Precedence
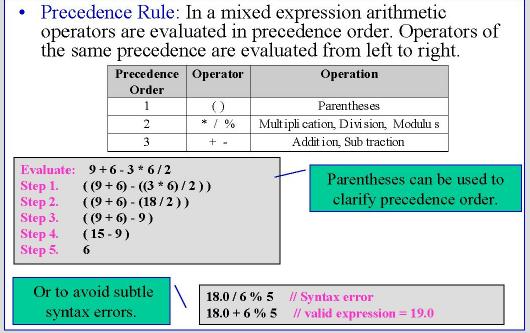
Increment/Decrement Operators
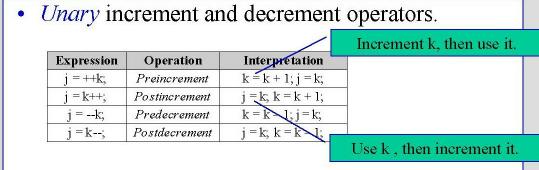
Java Language Rule:
- If ++k or --k occurs in an expression, k is incremented or
decremented before its value is used in the rest of the expression.
- If k++ or k-- occurs in an expression, k is incremented or
decremented after its value is used in the rest of the expression.
Shortcut Assignment Operators.
- Allows you to combine an assignment and arithmetic
in one operation.
- Can be used with integers or floating point.
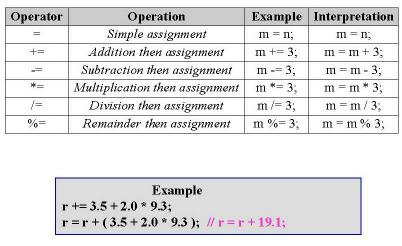
Review: Relational Operators
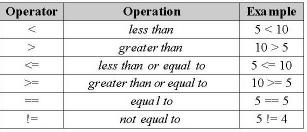
Note: = is an
assignment operator, while ==
is a relational operator.
Review: Numeric Operator Precedence
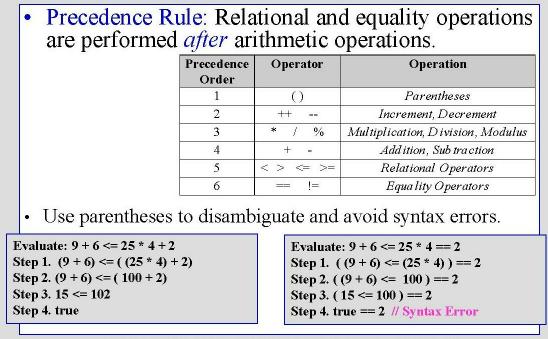
Case Study: Fahrenheit to Celsius
Design an applet that converts Fahrenheit to Celsius temperatures.
Note the following objects and interactions:
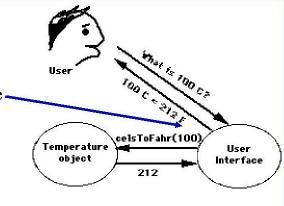
Problem Decomposition.
- 2 classes: Temperature (performs conversion) and TemperatureTest (interface
to user).
Temperature Class Design (data, methods, type of data).
- 2 public methods: one for each conversion (F to C, C to F)
- each takes an input parameter (double) and returns a result (double)
Implementation: Temperature
Class

Implementation: Testing and Debugging
It is important to develop good test data. For this application
the following tests would be appropriate:
- Test converting 0 degrees C to 32 degrees F.
- Test converting 100 degrees C to 212 degrees F.
- Test converting 212 degrees F to 100 degrees C.
- Test converting 32 degrees F to 0 degrees C.
These data test all the boundary conditions.
Testing Principle: The fact that your program
runs correctly on some data is no guarantee of its correctness. Testing reveals
the presence of errors, not their absence.
Implementation: TemperatureTest
Class
Purpose: Serve
as a user interface to Temperature class.
Algorithm Design:
- Input-process-output
- get a value from the user, calculate the result, display the result.
User Interface Design:
- Prompt the user - help guide the user's actions
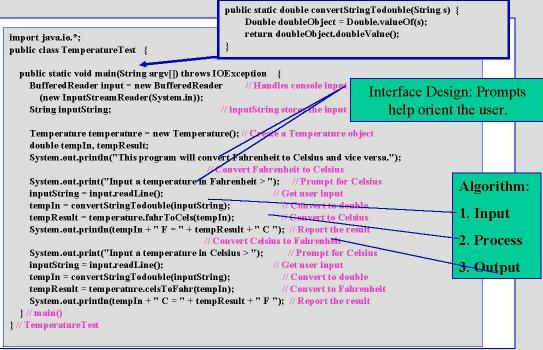
Implementation: Data Conversion
- The user enters data in string format, must be converted
to numeric for calculation.
- Method Composition: calling a method from another method. The output
from one becomes the input to the other.
public static Double valueOf(String);
public static doubleValue();
...
double d = Double.valueOf(inputString).doubleValue();
same as:
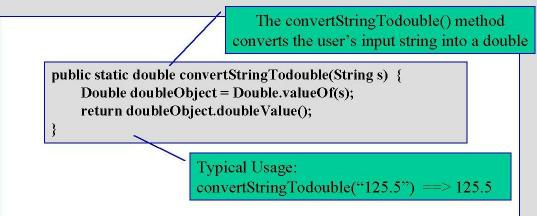
We can then just pass a string to the method and receive a numeric result,
without worrying about how the conversion is done.
Effective Design: Modularity Principle. Method
Abstraction.
- Encapsulating complex operations in a method
reduces the chances for error
- Also makes the program more readable and maintainable.
- Using methods raises the level of abstraction
in the program.
Design: TemperatureApplet
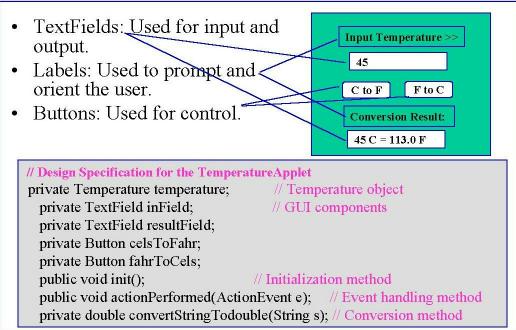
Implementation: TemperatureApplet (Data)
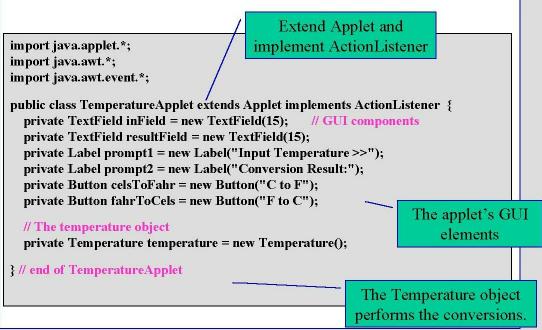
Implementation: TemperatureApplet (Methods)
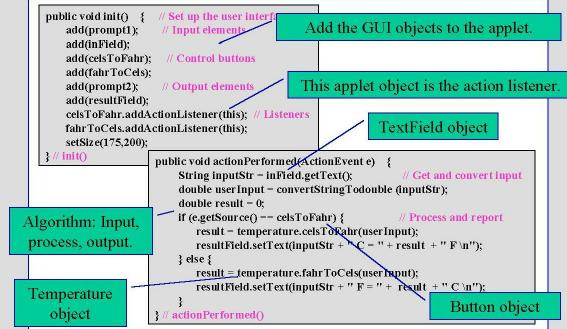
Integer-based CyberPet
Model (Data)
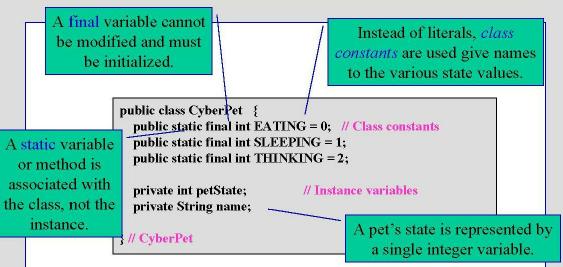
Maintainability Principle: Constants should be
used instead of literal values in a program to make the program easier to modify
and maintain.
Integer-based CyberPet Model (Constructors)
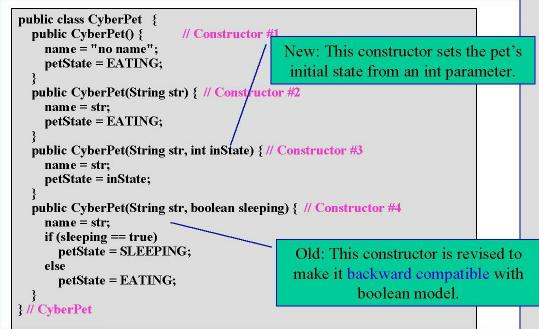
Integer-based CyberPet Model (Methods)
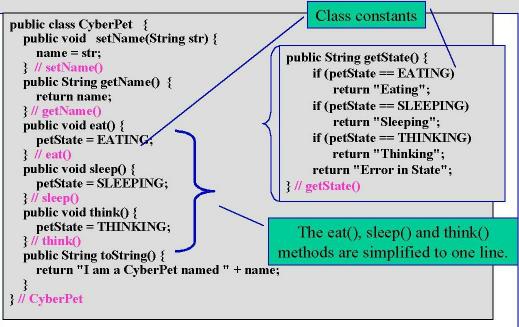
Advantages of the Integer-based CyberPet
Model
- Maintainability:
- One variable state representation makes it easier
to maintain.
- Robustness:
- Methods to change the state are simpler and less
error prone.
- Extensibility:
- Methods are self-contained making the model easier
to extend/modify.
- Modularity:
- A change in one method does not require changes
in other methods.
- Information Hiding:
- Design of its public interface and private elements
makes the new model compatible with the old.
Class Constants.
- Giving names to literal values
- A class constant is a final static variable associated with a class
- only one instance of it is stored, regardless of how many instances
of the class are created
- declared as public
- accessible (can be referenced) either from the class or its instances
- Benefits: readability, maintainability
- Convention: names in UPPER CASE
Review: Character Data and Operators
The char
type is a primitive data type.
- A character is represented by a 16-bit unsigned integer.
- A total of 216 = 65536 characters can be
represented.
- Java uses the international Unicode character
set.
- The first 128 Unicode characters are identical to the
characters in the 7-bit ASCII (American Standard Code for Information Interchange).
The ASCII Characters
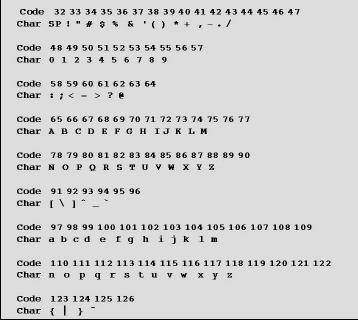
Character to Integer Conversions
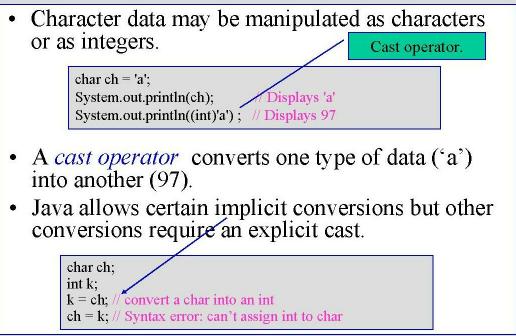
Type Conversions
- Java Rule: Java
permits implicit conversions from a narrower to a wider type.
- A cast operator must be used when converting a wider
into a narrower type.
- The cast operator can be used with any primitive type.
It applies to the variable or expression that immediately follows it.
Review: Relational Operators in Characters
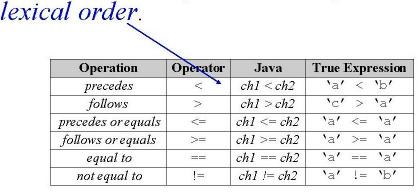
Example Character Conversions
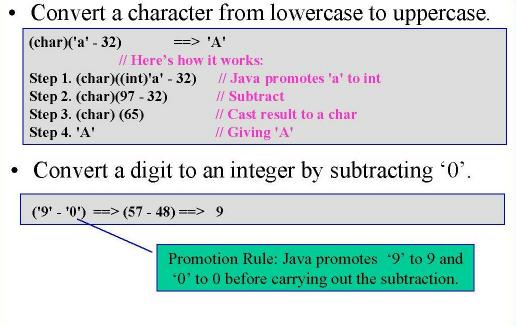
Conversion Methods
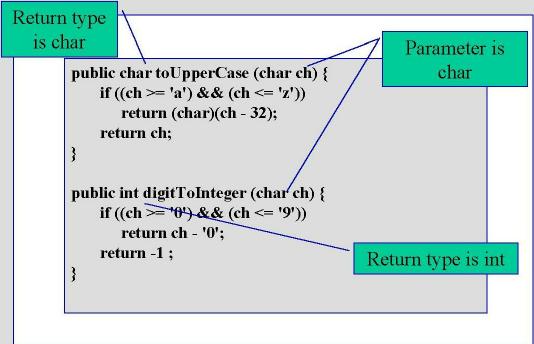
From the Java Library: java.lang.Math
Math Class Methods
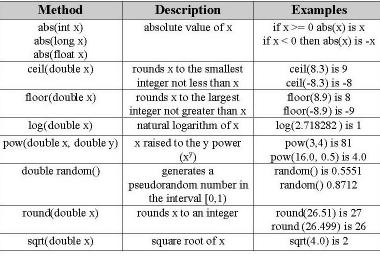
Math Class Example: Rounding
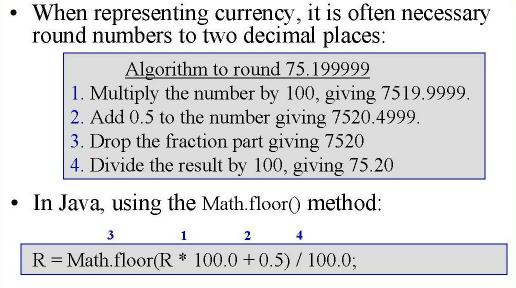
From the Java Library: NumberFormat
Example: Calculating Compound Interest
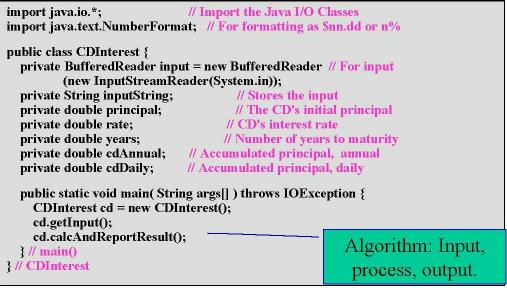
The CDInterest Application
CDInterest.getInput() Method
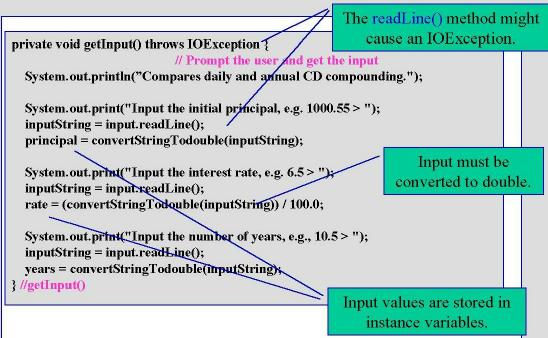
CDInterest.calcAndReportResult() Method
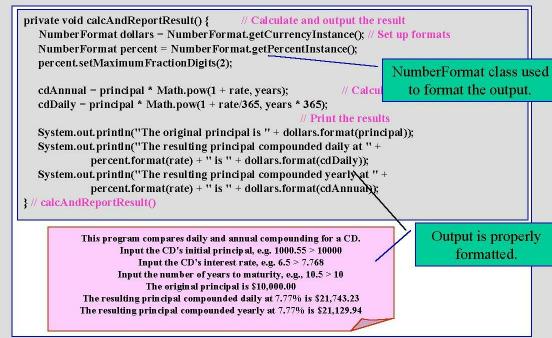
Leap Year Problem
Objectives:
- Design and writing a simple Java program.
- Use if-else and assignment statements.
- Use basic arithmetic and relational operators.
Leap Year Applet: Design Specification
- Decomposition: Classes
- LeapYearApplet: serves as the user interface.
- Year: decides whether a year is a leap year.
- Design: Year (modeled after Math class)
- Purpose: To determine if a year is a leap year
- Modifiers: Final, so it cannot be extended
- Constructor: Private, so cannot no instantiation
- Instance Variables: None (nothing to store)
- Public Instance Methods: None
- Public Static Methods: isLeapYear(int) tests whether
its parameter is a leap year.
- Design: LeapYearApplet
- Input Component: TextField
- Output: Use Applet.paint() method.
- Control Component: TextField can respond to actions
by registering with an ActionListener:
Review Exercises: #4, 6, 10, 12, 14