ISM 3230 Introduction to Programming
Chapter 7: Lists, Loops, and Printing
Chapter
7 Student Learning OBJECTIVES: |
Upon completion of this chapter,
you will be able to
- Create and use list boxes and combo boxes.
- Enter items into list boxes using the Properties window and
the AddItem method.
- Determine which item in a list is selected.
- Use the ListCount property to determine the number of items
in a list.
- Display a selected item from a list.
- Differentiate among the available types of combo boxes.
- Use Do/Loops and For/Next statements to iterate through a loop.
- Use the MsgBox function to determine the button pressed by the
user.
- Use the string functions Left, Right, and Mid, to refer to part
of a string, and use the Len function to count the number of characters
in a string.
- Send information to the printer using the Print method.
- Control the format of printing using commas, semicolons, the
Tab function, and the Spc function.
|
Download Example Programs
List Boxes and Combo Boxes
- List/Combo boxes allow a list of items
- Types include
- simple combo boxes
- dropdown combo boxes
- dropdown lists
- Combo box has style property; list does not
- Combo box has design-time text property; list box has only run-time
text property.
- Combo box does not have a MaxLength property; a list box does.
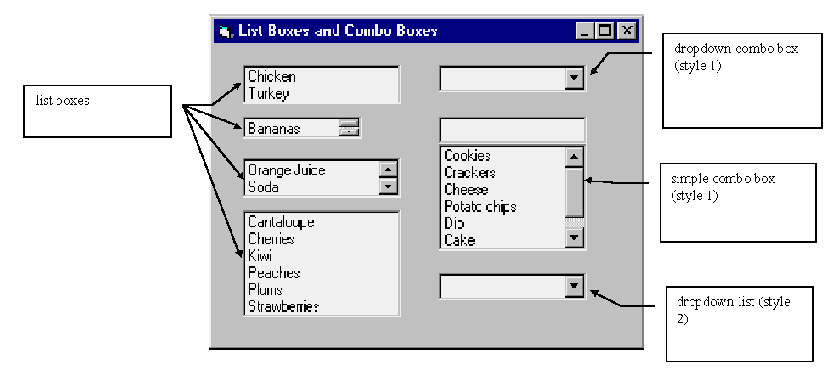
Filling the List
- You can fill the list at design- or run-time
- Fill at design time if list rarely changes
- Click List property, type entry, press Ctrl+Enter
- Make Sorted property true to keep list in order
- Fill at run time if list is volatile
- Use AddItem method to
add items
- Form: object.AddItem value [,Index]
- Example: lstFruits.AddItem "Apple"
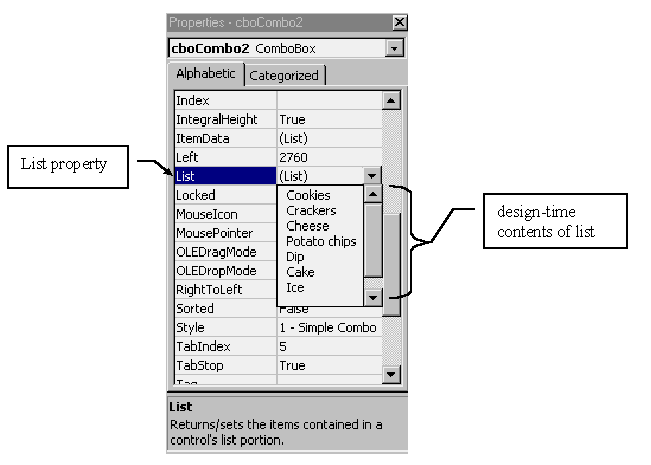
List/Combo Box Methods
- AddItem - Add item to list
cboFruits.AddItem "Kiwi"
cboFruits.AddItem cboFruits.Text
- Clear - Delete all items from the list
cboFruits.Clear
- Add items to a list with code like this:
lstSchools.AddItem "Stanford"
lstSchools.AddItem txtSchoolName.Text
lstSchools.AddItem cboFirstChoice.Text
- When a user types an entry in a combo box, that entry is not automatically
added to the list. Code must be invoked to add the item (on the lost focus
event, for example)
cboIdeaList.AddItem cboIdeaList.Text
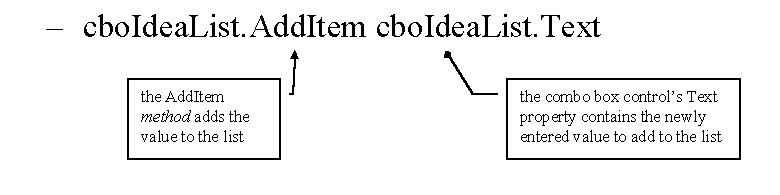
List/Combo Box Properties
- Sorted maintains list in order (if true)
- ListIndex holds user-selected item index number
- begins at zero, -1 if none selected
- setting the ListIndex property highlights a selection in the list
- ListCount is number of items in list
- if the list is empty, then the ListCount is zero
- List object.List(index) extracts string value of list item
- Deselect all items in a list by setting the ListIndex
property to -1
- Set the ListIndex property to 0 to select (highlight) the first item
in the list
- Remember that indexes run from 0 to n-1
- ListCount holds the number of items in a list.
- ListCount is always one higher than the highest ListIndex.
- Display one of the items in a list by selecting the list object and
referring to the ListIndex value as an index:
lstBookTypes(iValue)
List/Combo box Events
- Change
- Change event occurs whenever the user types anything into a combo
box (styles 0 and 1).
- Each keystroke generates another change event.
- A list box does not have a change event, because list boxes do
not have text boxes—only fixed lists that the user cannot add to by typing
at runtime.
- GotFocus
- GotFocus event occurs when a control—a list box or combo box
for example—receives control.
- LostFocus
- LostFocus event occurs as the control loses the focus—when the
user tabs to another control on the form for example.
Do Loops
- Allow repeated execution of instructions
- Do loop terminates on a condition you specify
- Do loop can test for condition at
- Top of loop (pretest)
- Bottom of loop (posttest)
- Loops can continue while a condition is either true or false
- Go while True: …While condition
- Go while False: …Until condition
- Be sure to change the loop variable within the loop or it will loop
infinitely!
For/Next Loops
- For/Next allows you to repeat series of instructions using a counter
(a loop index)
- Form:
For loopindex = initialvalue
To endvalue [Step k]
… body of loop …
Next [loopindex]
- You can loop "backwards" by using a negative increment
- Loop testing is done before executing body
- Do not change the loop control inside the body
- Halt infinite loops by pressing Ctrl+Break
- Exit For allows early For loop exit from
within the body, but should be avoided - poor programming practice
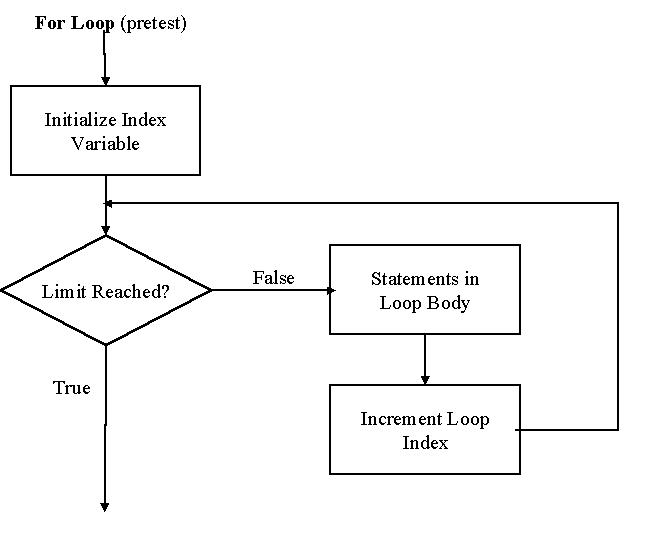
- Looping through a list:
Dim intLoopIndex As Integer
For intLoopIndex = 0 To lstSchools.ListCount
- 1
... (body of loop here)...
Next intLoopIndex
- Loop test is for greater than limit, not greater than or equal
to limit
- Use For/Next loop when you
know the number of times a loop will run
- When loop decrements, the test is for strictly less than the ending
value
- The For/Next ending condition
may exist before entering the loop. If so, then the loop body is skipped
- Example of an infinite (endless) loop:
Dim intLoop As Integer
For intLoop = 0 To 10 Step 1
intLoop = 1
Next
Using the MsgBox Function
- MsgBox has a function form (we have used statement form until now)
- MsgBox function returns which key the user pressed of those available
- Use VB intrinsic constants to determine which key was pressed.
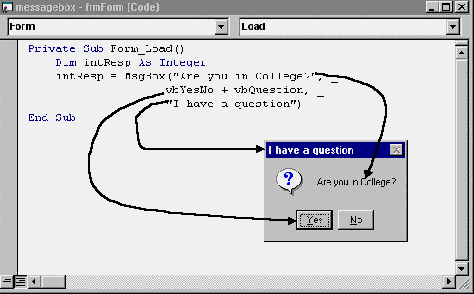
MsgBox Values
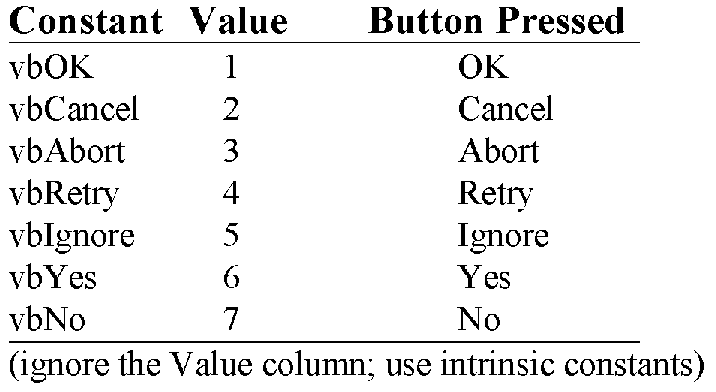
Important button and icon values:
- Button to Display Intrinsic Constant
- OK - vbOKOnly
- OK and Cancel - vbOKCancel
- Abort, Retry, Ignore - vbAbortRetryIgnore
- Yes, No, Cancel - vbYesNoCancel
- Yes and No - vbYesNo
- Retry and Cancel - vbRetryCancel
- Icons to Display Intrinsic Constant
- Critical Message - vbCritical
- Warning Query - vbQuestion
- Warning Message - vbExclamation
- Information Message - vbInformation
Examples of using instrinsic constants:
intResp = MsgBox(strMess, vbYesNoCancel
+ vbQuestion, "Do
What?")
intResp = MsgBox(strMess, vbCancel +
vbCritical, "Big
Problems")
intResp = MsgBox(strMess, vbOKOnly +
vbInformation, "FYI")
Using String Functions
- Left(str, nchars)
- extract left nchars
- Right(str, nchars)
- extract right nchars
- Mid(str,start,[nchars])
- get middle chars
- str is any string, nchars is integer, and start is integer
number of starting position
- Len(str) returns #
chars in string
- Suppose a text box named txtName contains the following:

- Left(txtName.Text, 3) is "Wha"
(without the quotation marks)
- Right(txtName.Text, 3) is "ob?"
(without the quotation marks)
- Mid(txtName.Text, 6, 5) is "about"
(without the quotation marks)
- Mid (txtname.Text, 6) is "about
Bob?" (without the quotation marks)
- String functions and looping facilitate the "zooming" feature of many Microsoft list boxes and combo boxes.
- "Zooming" causes a search of the list, refining the search with each additional keystroke the user enters.
Examine the following code to ensure yourself that it mimics the Windows activity
of "zooming" to a list box entry in attempting to match what the user
types:
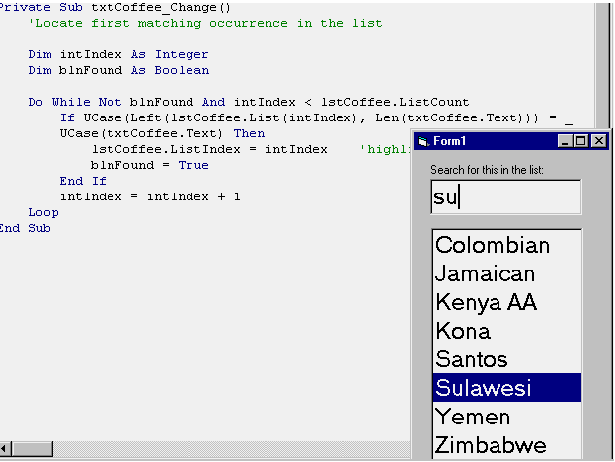
Sending Information to the Printer
- Visual Basic’s Print method applies to a Form, a printer object, the Debug
window, or a picture box
- Printer.Print — sends output to printer
- Print is a method of the Printer object
- Printing is somewhat difficult, because VB is designed for use with forms,
not printers
- You format printer lines just like normal Basic programming does—with commas,
print zones, Tab, Spc functions, etc.
- Examples:
Printer.Print, "Hello
World" ‘prints message in 2nd print zone
Printer.print "Name:";
txtName ‘do not use print zones
Printer.Print "" ‘print
a blank line
- Output is not actually printed until:
- job is terminated or
- you go to a new page (use the NewPage
method) or
- you end the entire printing process (use EndDoc
method)
- Change font: Printer.Font.Name
= "Times New Roman"
- Change point size: Printer.Font.Size
= 12
- Go to a new page: Printer.NewPage
- Send end command Printer.EndDoc
Formatting Lines
- Printer.print x, y, z prints in three "zones"
- Printer.print x;y;z ignores zones
- Trailing comma or semicolon causes next print method to continue the line
- Each .print starts on a new
line
- Printer.print
(alone) prints blank line
- Tab(20) advances to 20th
position:
Printer.Print Tab(20); "Name:"
- Spc(17) inserts spaces (17)
in line
- Numbers print with one space for sign of #
- Printer.NewPage sends page
to printer and goes to new page
- Printer.EndDoc sends page
to printer and halts print job
Printing to a Picture Box
- You can use Print method with a picture box to avoid actually printing a
result:
- picMyPictureBox.Print "howdy" prints to
a picture box
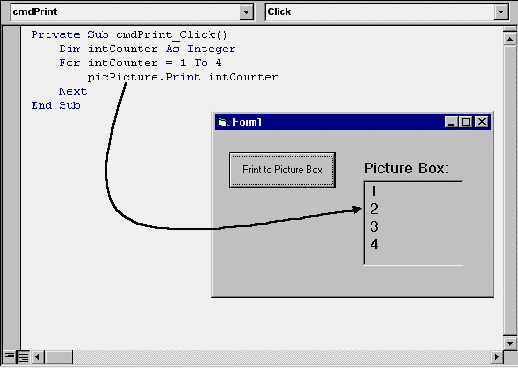
Hands-On Programming Example
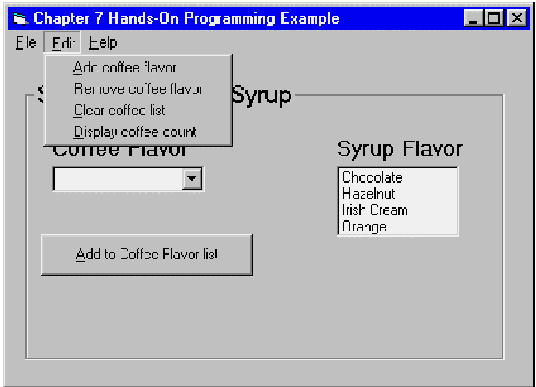
Chapter Summary:
OBJECTS
|
|
PROPERTIES
|
|
METHODS
|
|
STATEMENTS
|
|
FUNCTIONS
|
List box
|
|
List
|
|
AddItem
|
|
Do / Loop
|
|
Left
|
Combo box
|
|
ListCount
|
|
Clear
|
|
Exit For
|
|
Len
|
|
|
ListIndex
|
|
EndDoc
|
|
For / Next
|
|
Mid
|
|
|
Sorted
|
|
NewPage
|
|
|
|
Right
|
|
|
Style
|
|
Print
|
|
|
|
Spc
|
|
|
|
|
RemoveItem
|
|
|
|
Tab
|